You can host your web service on wamp server installed in your machine or you can simply use a cloud server like 000webhost.
1. To keep things simple Im going to use a dummy php code as follows
<?php
echo "Hello world!!!!"
?>
Create this php file and copy it in to www directory of wamp server or upload it in to a cloud server.2. Here is the code for Android activity
import android.app.Activity;
import android.os.Bundle;
import java.io.IOException;
import android.widget.TextView;
import org.apache.http.HttpResponse;
import org.apache.http.client.HttpClient;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.impl.client.DefaultHttpClient;
import org.apache.http.util.EntityUtils;
public class AndroidWebActivity extends Activity {
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
HttpClient httpclient = new DefaultHttpClient();
HttpPost httppost = new HttpPost("http://www.codeincloud.tk/First.php");
try {
HttpResponse response = httpclient.execute(httppost);
final String str = EntityUtils.toString(response.getEntity());
TextView tv = (TextView) findViewById(R.id.textView1);
tv.setText(str);
}
catch (IOException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
Note : If you are using wamp server dont use local host for the url in line 18, use 10.0.2.2.
Eg: http://10.0.2.2/android_connect/first.php
Dont forget to add internet permission.
Output of the program :
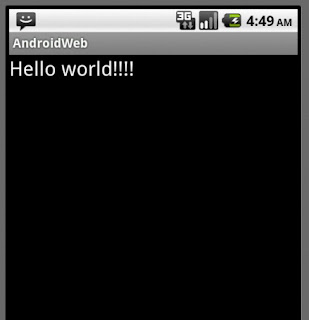